【个人使用】搭建一套规范的 Vue3 工程化项目
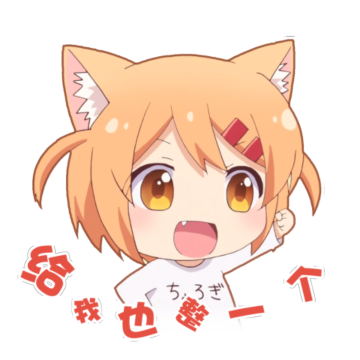
本文将使用 pnpm + vite 搭建一套拥有 prettier、ESLint、Stylelint、husky、lint-staged 以及提交规范的 vue3 + ts 项目
搭建一套规范的 Vue3 工程化项目
一、初始化项目
使用 pnpm 和 vite 初始化一个 vue3 + ts 项目
1 | pnpm create vite |
如果觉得下面步骤过于繁琐,可使用 cli 工具完成配置
1 | npm i -g sunshj |
执行 sun config
,如果是 pnpm monorepo 项目,需要使用 sun config -w
二、配置 Prettier
VS Code 需要安装 prettier 扩展
安装 prettier
1
pnpm i -D prettier
安装配置文件
1
pnpm i -D @sunshj/prettier-config
package.json
添加脚本和prettier
配置1
2
3
4
5
6{
"scripts": {
"format": "prettier --write ."
},
"prettier": "@sunshj/prettier-config"
}
三、配置 ESLint
VS Code 需要安装 ESLint 扩展并且开启
Use Flat Config
安装 ESLint
1
pnpm i -D eslint@8
安装配置文件
1
pnpm i -D @sunshj/eslint-config
根目录创建
eslint.config.js
配置文件1
2
3
4
5import { defineConfig } from '@sunshj/eslint-config'
export default defineConfig({
files: ['src/**/*.{vue,ts,js}'],
})package.json
添加脚本1
"lint": "eslint ."
四、配置 Stylelint
VS Code 需要安装 Stylelint 扩展 ,并进行配置
1 | { |
安装 Stylelint
1
pnpm i -D stylelint@16
安装配置文件
1
pnpm i -D @sunshj/stylelint-config
package.json
添加脚本和stylelint
配置1
2
3
4
5
6
7
8{
"scripts": {
"style:lint": "stylelint --cache --fix \"src/**/*.{vue,css,scss}\" --cache --cache-location node_modules/.cache/stylelint/"
},
"stylelint": {
"extends": "@sunshj/stylelint-config"
}
}
五、配置 husky 和 lint-staged
安装 husky 和 lint-staged
1
pnpm i -D husky@8 lint-staged@15
package.json
添加 lint-staged 配置1
2
3
4
5
6"lint-staged": {
"src/**/*.{vue,js,ts}": [
"eslint --fix",
"prettier --write"
]
}package.json
添加脚本1
"prepare": "husky install"
执行
pnpm prepare
添加 pre-commit 钩子
1
npx husky set .husky/pre-commit "npx lint-staged"
六、配置提交规范
安装 cz-git 、commitizen 以及 commitlint
1
pnpm i -D cz-git commitizen commitlint
建议全局安装
commitizen
,如此一来可以快速使用cz
或git cz
命令进行启动。package.json
添加 脚本和config
指定使用的适配器1
2
3
4
5
6
7
8
9
10{
"scripts": {
"commit": "git-cz"
},
"config": {
"commitizen": {
"path": "node_modules/cz-git"
}
}
}根目录创建
.commitlintrc.cjs
配置文件1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51/** @type {import('cz-git').UserConfig} */
module.exports = {
rules: {
'subject-empty': [2, 'never'],
},
prompt: {
messages: {
type: '选择你要提交的类型 :',
scope: '选择一个提交范围(可选):',
customScope: '请输入自定义的提交范围 :',
subject: '填写简短精炼的变更描述 :\n',
body: '填写更加详细的变更描述(可选)。使用 "|" 换行 :\n',
breaking: '列举非兼容性重大的变更(可选)。使用 "|" 换行 :\n',
footerPrefixesSelect: '选择关联issue前缀(可选):',
customFooterPrefix: '输入自定义issue前缀 :',
footer: '列举关联issue (可选) 例如: #31, #I3244 :\n',
confirmCommit: '是否提交或修改commit ?',
},
types: [
{ value: 'feat', name: 'feat: 新增功能 | A new feature' },
{ value: 'fix', name: 'fix: 修复缺陷 | A bug fix' },
{ value: 'docs', name: 'docs: 文档更新 | Documentation only changes' },
{
value: 'style',
name: 'style: 代码格式 | Changes that do not affect the meaning of the code',
},
{
value: 'refactor',
name: 'refactor: 代码重构 | A code change that neither fixes a bug nor adds a feature',
},
{ value: 'perf', name: 'perf: 性能提升 | A code change that improves performance' },
{
value: 'test',
name: 'test: 测试相关 | Adding missing tests or correcting existing tests',
},
{
value: 'build',
name: 'build: 构建相关 | Changes that affect the build system or external dependencies',
},
{
value: 'ci',
name: 'ci: 持续集成 | Changes to our CI configuration files and scripts',
},
{ value: 'revert', name: 'revert: 回退代码 | Revert to a commit' },
{
value: 'chore',
name: 'chore: 其他修改 | Other changes that do not modify src or test files',
},
],
},
}添加 commit-msg 钩子
1
npx husky set .husky/commit-msg "npx --no-install commitlint --config .commitlintrc.cjs --edit $1"
提交执行
pnpm commit
即可
评论